目次 |
---|
はじめに
前回は、スライド内のオブジェクト(Shapeなど)を取得してみました。
今回は、さらにもう一歩踏み込んで、Shapeの属性値を変更してみます。
今回は、前回の雛型ファイル(.pptx)をそのまま使用します。
属性値の変更はどうすればよいか
前回は、オブジェクトの属性値を取得しました。
その属性値を変更するにはどうすればよいかというと、単に属性に値を設定してあげれば変更できます。
例えば、属性値を取得する場合は、
float f = オブジェクト.Width; |
といった感じでしたが、属性値を設定する場合は、
オブジェクト.Width = 123.45; |
のようにすれば、属性値の変更ができます。
フォームの改造
今回も、前回作ったフォームを少し改造してみます。
今回は、以下のようなフォームにしました。
改造点は、ログ表示領域を少し小さくして下に下げたことと、オブジェクトの属性変更・保存ボタンを追加し、属性値の表示と値の変更ができるテキストボックスを追加したことです。
コードの追加
今回のコードの追加は、次のように行います。
まず、前回の「2.ページ内オブジェクトの取得」ボタンを押したときに、処理の初めに同時にコンボボックスをクリアし、オブジェクトの情報を取得するループ内でオブジェクトの名称を取得してコンボボックスに追加します。
また、プログラムが長く分かりにくくなってきたので、メソッドコメントも追加しました。
// [★属性変更用]Comboboxの項目をクリアします。 comboBox1.Items.Clear();
// [★属性変更用]取得した名称をComboboxに追加します。 comboBox1.Items.Add(s.Name);
さらに、コンボボックスの選択状態が変わったときに、オブジェクトの属性値の取得を行います。
今回は、お試しプログラムにつき、作りがよくないですが、コンボボックスの選択状態が変わるたびに雛型ファイルを読んで、オブジェクトの属性値の取得を行っています。
/// <summary> /// 属性変更用コンボボックスの選択状態が変わった時の処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) { int idx = comboBox1.SelectedIndex; string name = comboBox1.Items[idx].ToString(); var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); // 属性値(textbox)をクリアします。 label4.Text = ""; textBox4.Text = ""; textBox5.Text = ""; textBox6.Text = ""; textBox7.Text = ""; textBox8.Text = ""; // 1ページ内のShapeについてプロパティ値を取得し、comboboxで選択したオブジェクトの属性を抽出します。 foreach (PPt.Shape s in file.Slides[1].Shapes) { if (s.Name.Equals(name)) { label4.Text = s.AutoShapeType.ToString(); textBox4.Text = s.Width.ToString(); textBox5.Text = s.Height.ToString(); textBox6.Text = s.Top.ToString(); textBox7.Text = s.Left.ToString(); // テキストがあるオブジェクトについては、テキストを取得します。 if (s.TextFrame.HasText == MsoTriState.msoTrue) { textBox8.Text = s.TextFrame.TextRange.Text; } textBox2.AppendText("Shape[" + name + "]の属性を取得しました。\n"); break; } } textBox2.AppendText("ファイルをクローズしました。\n"); }
また、「3.オブジェクトの属性変更・保存」ボタンが押されたときに、オブジェクトの属性値を変更した上で、雛型ファイルを別ファイルに保存する処理を追加しました。
/// <summary> /// 「3.オブジェクトの属性変更・保存」ボタンを押したときの処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void button6_Click(object sender, EventArgs e) { var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); int idx = comboBox1.SelectedIndex; string name = comboBox1.Items[idx].ToString(); foreach (PPt.Shape s in file.Slides[1].Shapes) { if (s.Name.Equals(name)) { try { s.Width = float.Parse(textBox4.Text); s.Height = float.Parse(textBox5.Text); s.Top = float.Parse(textBox6.Text); s.Left = float.Parse(textBox7.Text); } catch { textBox2.AppendText("属性値に誤りがあります。\n"); file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); return; } // テキストがあるオブジェクトについては、テキストを取得します。 if (s.TextFrame.HasText == MsoTriState.msoTrue) { s.TextFrame.TextRange.Text = textBox8.Text; } textBox2.AppendText("Shape[" + name + "]の属性を変更しました。\n"); break; } } file.SaveAs(textBox3.Text); textBox2.AppendText("別ファイル(" + textBox3.Text + ")に保存しました。\n"); file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); }
すべてのコードは以下のようになります。
using System; using System.Windows.Forms; using PPt = Microsoft.Office.Interop.PowerPoint; using Microsoft.Office.Core; namespace PptEditTest01 { public partial class Form1 : Form { /// <summary> /// 初期処理です。 /// </summary> public Form1() { InitializeComponent(); } /// <summary> /// 雛型ファイルの「参照」ボタンをクリックしたときの処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void button1_Click(object sender, EventArgs e) { OpenFileDialog ofd = new OpenFileDialog(); ofd.FileName = ""; ofd.InitialDirectory = @"C:\"; ofd.Filter = "PowerPointファイル(*.pptx)|*.pptx|すべてのファイル(*.*)|*.*"; ofd.Title = "開くファイルを選択してください"; ofd.RestoreDirectory = true; if (ofd.ShowDialog() == DialogResult.OK) { textBox1.Text = ofd.FileName; } } /// <summary> /// 「1.ページの複製」ボタンをクリックしたときの処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void button2_Click(object sender, EventArgs e) { var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); file.Slides[1].Duplicate();// スライド1をコピー textBox2.AppendText("1ページ目のスライド2ページ目にコピーしました。\n"); file.SaveAs(textBox3.Text); textBox2.AppendText("別ファイル(" + textBox3.Text + ")に保存しました。\n"); file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); } /// <summary> /// 「クリア」ボタンをクリックしたときの処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void button3_Click(object sender, EventArgs e) { textBox2.Clear(); } /// <summary> /// 作成ファイルの「参照」ボタンをクリックしたときの処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void button4_Click(object sender, EventArgs e) { OpenFileDialog ofd = new OpenFileDialog(); ofd.FileName = "Output.pptx"; ofd.InitialDirectory = @"C:\"; ofd.Filter = "PowerPointファイル(*.pptx)|*.pptx|すべてのファイル(*.*)|*.*"; ofd.Title = "保存するファイル名を指定してください"; ofd.RestoreDirectory = true; ofd.CheckFileExists = false; if (ofd.ShowDialog() == DialogResult.OK) { textBox3.Text = ofd.FileName; } } /// <summary> /// 「2.ページ内オブジェクトの取得」ボタンをクリックしたときの処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void button5_Click(object sender, EventArgs e) { // [★属性変更用]Comboboxの項目をクリアします。 comboBox1.Items.Clear(); var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); int cnt = 1; // 1ページ内のShapeについてプロパティ値を取得します。 foreach (PPt.Shape s in file.Slides[1].Shapes) { textBox2.AppendText("Shape[" + cnt + "] Name:" + s.Name + "\n"); textBox2.AppendText("Shape[" + cnt + "] AutoShapeType:" + s.AutoShapeType.ToString() + "\n"); textBox2.AppendText("Shape[" + cnt + "] Width:" + s.Width + "\n"); textBox2.AppendText("Shape[" + cnt + "] Height:" + s.Height + "\n"); textBox2.AppendText("Shape[" + cnt + "] Top:" + s.Top + "\n"); textBox2.AppendText("Shape[" + cnt + "] Left:" + s.Left + "\n"); // テキストがあるオブジェクトについては、テキストを取得します。 if (s.TextFrame.HasText == MsoTriState.msoTrue) { textBox2.AppendText("Shape[" + cnt + "] TextFrame.TextRange.Text:" + s.TextFrame.TextRange.Text + "\n"); } // [★属性変更用]取得した名称をComboboxに追加します。 comboBox1.Items.Add(s.Name); cnt++; } file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); } /// <summary> /// 属性変更用コンボボックスの選択状態が変わった時の処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) { int idx = comboBox1.SelectedIndex; string name = comboBox1.Items[idx].ToString(); var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); // 属性値(textbox)をクリアします。 label4.Text = ""; textBox4.Text = ""; textBox5.Text = ""; textBox6.Text = ""; textBox7.Text = ""; textBox8.Text = ""; // 1ページ内のShapeについてプロパティ値を取得し、comboboxで選択したオブジェクトの属性を抽出します。 foreach (PPt.Shape s in file.Slides[1].Shapes) { if (s.Name.Equals(name)) { label4.Text = s.AutoShapeType.ToString(); textBox4.Text = s.Width.ToString(); textBox5.Text = s.Height.ToString(); textBox6.Text = s.Top.ToString(); textBox7.Text = s.Left.ToString(); // テキストがあるオブジェクトについては、テキストを取得します。 if (s.TextFrame.HasText == MsoTriState.msoTrue) { textBox8.Text = s.TextFrame.TextRange.Text; } textBox2.AppendText("Shape[" + name + "]の属性を取得しました。\n"); break; } } textBox2.AppendText("ファイルをクローズしました。\n"); } /// <summary> /// 「3.オブジェクトの属性変更・保存」ボタンを押したときの処理です。 /// </summary> /// <param name="sender">イベント送信元</param> /// <param name="e">イベント</param> private void button6_Click(object sender, EventArgs e) { var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); int idx = comboBox1.SelectedIndex; string name = comboBox1.Items[idx].ToString(); foreach (PPt.Shape s in file.Slides[1].Shapes) { if (s.Name.Equals(name)) { try { s.Width = float.Parse(textBox4.Text); s.Height = float.Parse(textBox5.Text); s.Top = float.Parse(textBox6.Text); s.Left = float.Parse(textBox7.Text); } catch { textBox2.AppendText("属性値に誤りがあります。\n"); file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); return; } // テキストがあるオブジェクトについては、テキストを取得します。 if (s.TextFrame.HasText == MsoTriState.msoTrue) { s.TextFrame.TextRange.Text = textBox8.Text; } textBox2.AppendText("Shape[" + name + "]の属性を変更しました。\n"); break; } } file.SaveAs(textBox3.Text); textBox2.AppendText("別ファイル(" + textBox3.Text + ")に保存しました。\n"); file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); } } }
スライド内オブジェクトの属性変更
では実際にページ内オブジェクトの属性変更を行ってみます。
実行結果は、以下のようになりました。
操作方法は、
1.雛型ファイルを設定
2.作成ファイルを設定
3.「2.ページ内オブジェクトの取得」をクリック
4.属性名のコンボボックスで、属性値の変更対象のオブジェクトを選択
5.属性値を書き換える
6.「3.オブジェクトの属性変更・保存」ボタンをクリック
という流れになります。
属性値変更の結果
属性値の変更を実際にやってみた結果は以下の通りです。
選択した属性値(座標・大きさ・テキスト)が変わっていますね。
変更前
変更後
まとめ
今回は、スライド内のオブジェクトの属性値の変更をしてみました。
ここまでできれば、雛型のPptxファイルを作ってShapeをテキストなどで特定してプロパティ値を変更すれば、様々な編集ができるようになりますね。
次回は、実際にこのような方法を応用して、何か使えるツールを作ってみたいと思います。
関連記事
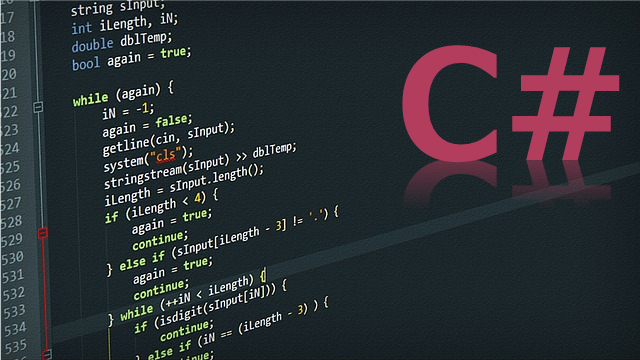
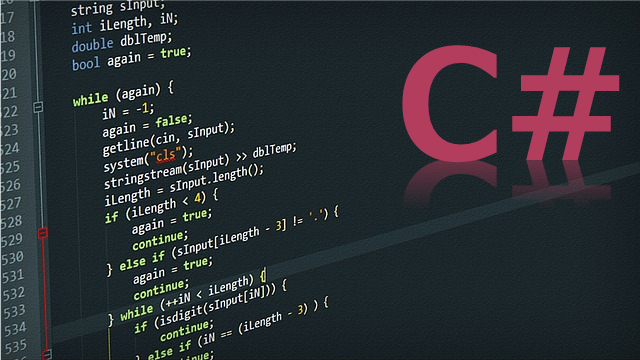
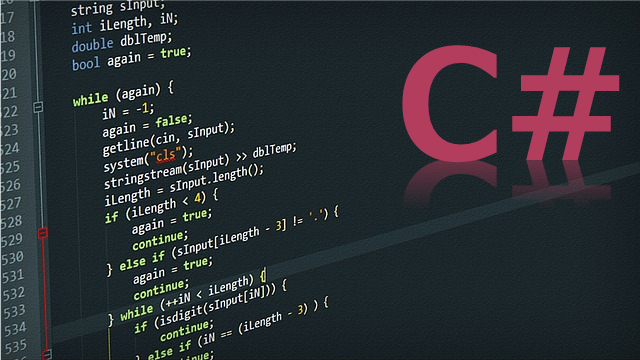