目次 |
---|
はじめに
前回は、お試し的にC#でPowerPointのファイルを開いてスライドを複製してみました。
今回は、もう一歩踏み込んで、スライド内のオブジェクト(Shapeなど)を取得してみます。
雛型ファイル(.pptx)の用意
まずは、前回と同様に雛型ファイルを用意します。
今回は、以下のようにいくつかのオブジェクトを適当に配置しました。
どんな情報が取得できるかわからないので、とりあえずテキストが設定できるものはテキストを入れておきました。
フォームの改造
前回作ったフォームを少し改造してみます。
今回は、以下のようなフォームにしました。
改造点は、フォームサイズを縦長にしたことと、オブジェクトの情報を取得するボタンを追加し、ログ表示領域を大きくしたことです。
コードの追加
前回のコードに対して、以下のコードを追加しました。
まず
using PPt = Microsoft.Office.Interop.PowerPoint; |
を追加しました。これでPowerPointに関する各種のクラスやインターフェース情報をPPt使って参照できます。そして、追加したボタンのイベントのところに、以下のコードを追加しました。
var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); int cnt = 1; // 1ページ内のShapeについてプロパティ値を取得します。 foreach(PPt.Shape s in file.Slides[1].Shapes) { textBox2.AppendText("Shape["+cnt+"] Name:" + s.Name+"\n"); textBox2.AppendText("Shape[" + cnt + "] AutoShapeType:" + s.AutoShapeType.ToString() + "\n"); textBox2.AppendText("Shape[" + cnt + "] Width:" + s.Width + "\n"); textBox2.AppendText("Shape[" + cnt + "] Height:" + s.Height + "\n"); textBox2.AppendText("Shape[" + cnt + "] Top:" + s.Top + "\n"); textBox2.AppendText("Shape[" + cnt + "] Left:" + s.Left + "\n"); // テキストがあるオブジェクトについては、テキストを取得します。 if(s.TextFrame.HasText==MsoTriState.msoTrue) { textBox2.AppendText("Shape[" + cnt + "] TextFrame.TextRange.Text:" + s.TextFrame.TextRange.Text + "\n"); } cnt++; } file.Close(); textBox2.AppendText("ファイルをクローズしました。\n");
処理内容としては、1ページめのスライドのすべてのShapeオブジェクトについて、プロパティ値を取得してログ表示領域に出力する、というものです。
すべてのコードは、以下のようになります。
using System; using System.Windows.Forms; using PPt = Microsoft.Office.Interop.PowerPoint; using Microsoft.Office.Core; namespace PptEditTest01 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { OpenFileDialog ofd = new OpenFileDialog(); ofd.FileName = ""; ofd.InitialDirectory = @"C:\"; ofd.Filter = "PowerPointファイル(*.pptx)|*.pptx|すべてのファイル(*.*)|*.*"; ofd.Title = "開くファイルを選択してください"; ofd.RestoreDirectory = true; if (ofd.ShowDialog() == DialogResult.OK) { textBox1.Text = ofd.FileName; } } private void button2_Click(object sender, EventArgs e) { var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); file.Slides[1].Duplicate();// スライド1をコピー textBox2.AppendText("1ページ目のスライド2ページ目にコピーしました。\n"); file.SaveAs(textBox3.Text); textBox2.AppendText("別ファイル("+ textBox3.Text+ ")に保存しました。\n"); file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); } private void button3_Click(object sender, EventArgs e) { textBox2.Clear(); } private void button4_Click(object sender, EventArgs e) { OpenFileDialog ofd = new OpenFileDialog(); ofd.FileName = "Output.pptx"; ofd.InitialDirectory = @"C:\"; ofd.Filter = "PowerPointファイル(*.pptx)|*.pptx|すべてのファイル(*.*)|*.*"; ofd.Title = "保存するファイル名を指定してください"; ofd.RestoreDirectory = true; ofd.CheckFileExists = false; if (ofd.ShowDialog() == DialogResult.OK) { textBox3.Text = ofd.FileName; } } private void button5_Click(object sender, EventArgs e) { var ppt = new PPt.Application(); var pres = ppt.Presentations; var file = pres.Open(textBox1.Text, MsoTriState.msoFalse, MsoTriState.msoFalse, MsoTriState.msoFalse); textBox2.AppendText("pptxファイルをオープンしました。\n"); int cnt = 1; // 1ページ内のShapeについてプロパティ値を取得します。 foreach(PPt.Shape s in file.Slides[1].Shapes) { textBox2.AppendText("Shape["+cnt+"] Name:" + s.Name+"\n"); textBox2.AppendText("Shape[" + cnt + "] AutoShapeType:" + s.AutoShapeType.ToString() + "\n"); textBox2.AppendText("Shape[" + cnt + "] Width:" + s.Width + "\n"); textBox2.AppendText("Shape[" + cnt + "] Height:" + s.Height + "\n"); textBox2.AppendText("Shape[" + cnt + "] Top:" + s.Top + "\n"); textBox2.AppendText("Shape[" + cnt + "] Left:" + s.Left + "\n"); // テキストがあるオブジェクトについては、テキストを取得します。 if(s.TextFrame.HasText==MsoTriState.msoTrue) { textBox2.AppendText("Shape[" + cnt + "] TextFrame.TextRange.Text:" + s.TextFrame.TextRange.Text + "\n"); } cnt++; } file.Close(); textBox2.AppendText("ファイルをクローズしました。\n"); } } }
スライド内オブジェクトの取得
では実際にページ内オブジェクトの取得を行ってみます。
実行結果は、以下のようになりました。
この出力結果は、以下の通りでした。
pptxファイルをオープンしました。 Shape[1] Name:TextBox 3 Shape[1] AutoShapeType:msoShapeRectangle Shape[1] Width:193 Shape[1] Height:29.08126 Shape[1] Top:41 Shape[1] Left:69.66669 Shape[1] TextFrame.TextRange.Text:テキストボックス Shape[2] Name:Rectangle 4 Shape[2] AutoShapeType:msoShapeRectangle Shape[2] Width:113.5384 Shape[2] Height:34.61535 Shape[2] Top:87.64102 Shape[2] Left:69.66669 Shape[2] TextFrame.TextRange.Text:矩形 Shape[3] Name:Oval 1 Shape[3] AutoShapeType:msoShapeOval Shape[3] Width:113 Shape[3] Height:36 Shape[3] Top:153.3333 Shape[3] Left:69.66669 Shape[3] TextFrame.TextRange.Text:円/楕円 Shape[4] Name:Isosceles Triangle 2 Shape[4] AutoShapeType:msoShapeIsoscelesTriangle Shape[4] Width:112 Shape[4] Height:50.25646 Shape[4] Top:220.4102 Shape[4] Left:71.20512 Shape[4] TextFrame.TextRange.Text:三角 Shape[5] Name:Right Arrow 5 Shape[5] AutoShapeType:msoShapeRightArrow Shape[5] Width:92.53842 Shape[5] Height:62.66669 Shape[5] Top:306.1539 Shape[5] Left:79.89748 Shape[5] TextFrame.TextRange.Text:右矢印 Shape[6] Name:Down Arrow 6 Shape[6] AutoShapeType:msoShapeDownArrow Shape[6] Width:160 Shape[6] Height:46.66669 Shape[6] Top:380.9743 Shape[6] Left:47.20512 Shape[6] TextFrame.TextRange.Text:下矢印 Shape[7] Name:Straight Connector 8 Shape[7] AutoShapeType:msoShapeMixed Shape[7] Width:101.3334 Shape[7] Height:61.91874 Shape[7] Top:95.82481 Shape[7] Left:297.3333 Shape[8] Name:Straight Arrow Connector 10 Shape[8] AutoShapeType:msoShapeMixed Shape[8] Width:124 Shape[8] Height:85.74362 Shape[8] Top:220.4102 Shape[8] Left:325.3333 Shape[9] Name:Picture 7 Shape[9] AutoShapeType:msoShapeRectangle Shape[9] Width:146.6831 Shape[9] Height:146.6831 Shape[9] Top:116.599 Shape[9] Left:562.6583 ファイルをクローズしました。
各Shapeには、それぞれの形状に応じたAutoShapeTypeが指定されています。
Shapeには、下図のように多くの種類がありますが、それぞれ異なるAutoShapeTypeになっていると思います。(全部やっていないので憶測ですが)
なお、画像を1枚貼っていましたが、これは「msoShapeRectangle」となっているようです。単なるテキストも同じみたいですね。
ここまでで、Shapeの情報取得ができることがわかりました。
まとめ
今回は、スライド内のオブジェクト(Shapeなど)を取得してみました。
ここまでできれば、Shapeをテキストなどで特定してプロパティ値を変更すれば、様々な編集ができるようになりそうですね。
次回は、Shapeの属性変更など、実際の編集を行ってみたいと思います。
関連記事
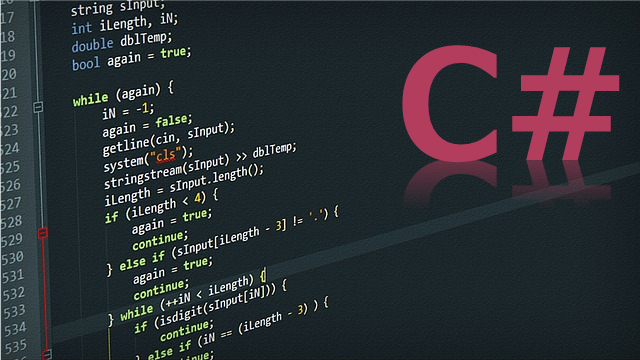
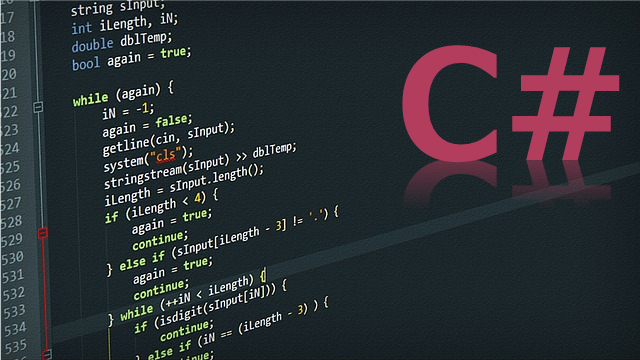
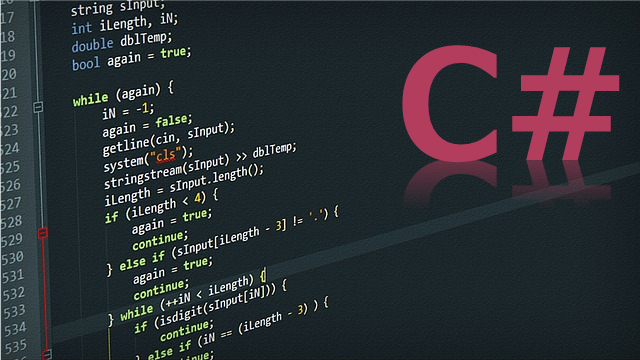